With a little knowledge of JavaScript, it is possible to programmatically create additional values and metrics from the existing data of an activity. Tredict calls this "custom fields".
Your self-created activity metrics are then available as usual in the training detail view, in the annual calendar view and in the evaluation and statistics.
This way you can easily implement your own ideas, create experimental metrics or extend your training with values you are used to from other analysis platforms.
In the glossary you will find a list of basic values of an activity that you can use.
Example I: Calculate the temperature difference of the watch with the outside temperature
Wrist temperature measurement has been standard on modern sports watches from Garmin, Suunto, Polar and others for a few years now.
If you now compare the temperature on the wrist with the true outdoor temperature from the weather service and look at the temperature difference, you can draw an interesting conclusion about the possible applications and accuracy of the temperature sensor of the watch.
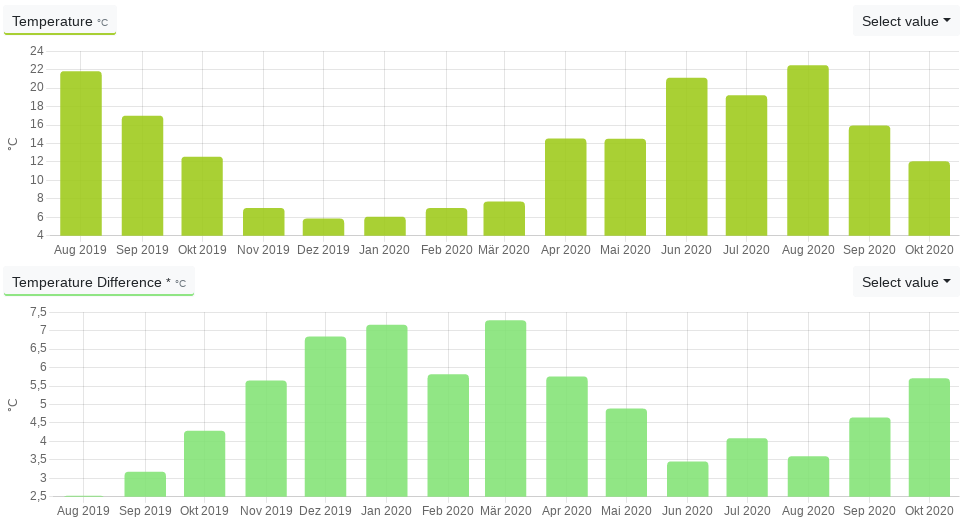
The calculated temperature difference between the device temperature and the outside temperature over the year at running activities.
In the aggregation chart, we can see that our calculated temperature difference when running in the cold season is 7 degrees Celsius, which is significantly higher than in the warm season.
Our custom field suggests that the temperature displayed by the clock can be treated as device temperature, but must not be used as outdoor temperature, because the difference is huge.
It would now be interesting to do the same with open water swimming activities. So it would probably be possible to determine the difference between water temperature and air temperature.
How do I create a custom field?
In the "Settings" category you will find the "Custom fields" subcategory.
Provided your running watch supports wrist temperature measurement, by looking at the activity metrics list, you will find the values temperature
and temperatureStation
.
From the outside temperature measured by the weather station and the temperature measured by the watch, you form the difference and return it.
The JavaScript code for calculating the temperature difference could not be simpler:
return this.temperature - this.temperatureStation;
Where this
is the object of the activity.
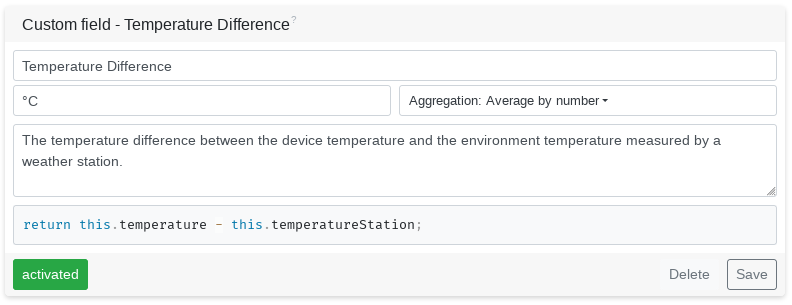
The custom field "Temperature Difference" is formed from the temperature measured by the sports watch and the temperature of the weather station.
For a more detailed explanation of creating custom metrics, see the glossary.
Note when creating the custom field that it can only return a number. It is not possible to display strings.
Example II: Replicate metrics from TrainingPeaks®
If you are used to metrics like Training Stress Score® (TSS®), Intensity Factor® (IF®) or the Variability Index (VI) from TrainingPeaks®, you can also replicate them in Tredict using the "Custom Fields" feature.
The formulas for this are explained on the page of TrainingPeaks and Tredict provides all the necessary baseline values at the activity to calculate the metrics.
The value powerPerceived
at a Tredict activity is to be used analogous to the Normalized Power® (NP®).
Note that there can be differences to all metrics, since TrainingPeaks may calculate these values differently internally and Tredict is not related to TrainingPeaks.
The JavaScript code to replicate the Training Stress Score® by power could look like this:
const { powerPerceived, ftp, duration } = this;
if (!powerPerceived || !ftp || !duration) {
return null;
}
const intensity = powerPerceived / ftp;
const tss = (duration * powerPerceived * intensity / (ftp * 3600)) * 100;
return Math.round(tss);
Disclaimer: TSS, NP, IF and TrainingPeaks are a registered trademark of Peaksware, LLC. Tredict does not claim to support the mentioned metrics directly. It is up to the user to decide whether to implement the mentioned metrics as a "Custom Field" using the known formulas, and the user is responsible for verifying their correctness.
Example III: Positive or negative split of an activity?
This example is a bit more complicated programmatically, as we will be using the sampled series data to determine if the activity has a positive or negative split.
The series data is only provided in the training detail view. Due to the amount of data in the series, it is not possible to load it in the statistics for each activity. So, unfortunately, this field is not displayed in the evaluation and analysis, but only in the activity detail view.
The "split" refers to the division exactly in the middle of the workout. A negative split is when the second half of the workout was run, cycled or swam faster. In contrast, the positive split shows you a slowing down in the second half.
Training theory favors the negative split because it is statistically more common when world-class athletes compete. Why this is so, certainly has several simultaneous causes. The negative split should not be a forced training goal, but only a secondary result and can thus be consulted as an indicator.
The code for calculating the negative split looks like this:
const { distance: totalDistance } = this;
const { data, sampleSize, endOffset } = this.seriesSampled || {};
const { distance } = data;
if (totalDistance == null || distance == null || !distance.length) {
return null;
}
const durationByLength = distance.length * sampleSize + endOffset;
const splitDuration = durationByLength / 2;
const splitDistance = distance[Math.round(splitDuration / sampleSize)];
const firstSplitPace = splitDuration / splitDistance;
const secondSplitPace = splitDuration / ((totalDistance / 1000) - splitDistance);
return firstSplitPace > secondSplitPace && 1 || 0;
In the future, it could be that the negative split will be implemented as a fixed precalculated field in Tredict. :-)